Program Overview
This advanced iOS development curriculum is tailored for professionals with 3-6 years of experience seeking to enhance their skills and advance to senior positions. The program builds on existing knowledge of Swift and iOS fundamentals to explore advanced topics, architectural patterns, and industry best practices used in production-level applications.
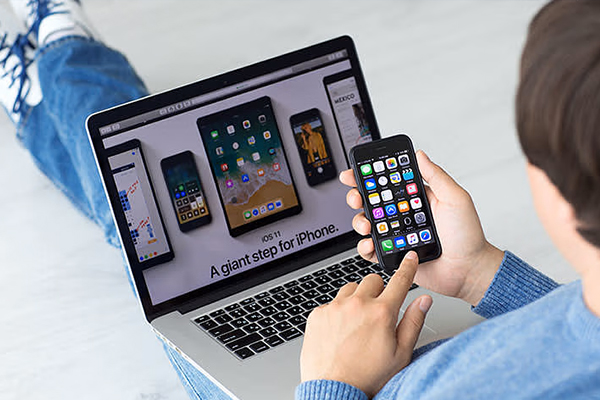
Detailed Curriculum
Module 1: Advanced Swift & Best Practices (4 hours)
- Swift Language Mastery
- Protocol-oriented programming techniques
- Functional programming in Swift
- Advanced generics and associated types
- Type erasure and type casting patterns
- Performance Optimization
- Memory management deep dive
- Value types vs reference types optimization
- Compile time vs runtime considerations
- Swift Evolution
- Latest Swift features and APIs
- Backward compatibility strategies
- Future Swift roadmap discussion
Module 2: Architectural Patterns (5 hours)
- MVC Evolution
- Problems with traditional MVC
- Effective controller management
- Avoiding massive view controllers
- MVVM Implementation
- Data binding techniques
- ViewModel design principles
- Testability advantages
- VIPER Architecture
- Component responsibilities
- Communication protocols
- Module boundaries and interfaces
- Other Architectures
- Clean Architecture approach
- Coordinator pattern
- Reactive architectures (RxSwift/Combine)
- Choosing the Right Architecture
- Project size considerations
- Team structure compatibility
- Migration strategies
Module 3: Advanced UI & Animations (5 hours)
- Custom UI Components
- Drawing with Core Graphics
- Advanced Auto Layout techniques
- Compositional layout with UICollectionView
- Responsive Interfaces
- Adaptive layouts across devices
- Dark mode implementation
- Accessibility considerations
- Advanced Animations
- UIKit animations vs Core Animation
- Interactive transitions
- Gesture-driven interfaces
- Physics-based animations
- SwiftUI Integration
- Mixing UIKit and SwiftUI
- Porting UIKit components to SwiftUI
- Shared state management
Module 4: Concurrency & Multithreading (4 hours)
- Threading Models
- GCD deep dive
- Operation queues and dependencies
- Thread safety patterns
- Swift Concurrency
- Async/await implementation
- Structured concurrency
- Task management and cancellation
- Performance Optimization
- Race condition prevention
- Deadlock detection and resolution
- Background processing techniques
- Practical Applications
- Image processing pipelines
- Background data sync
- Long-running operations management
Module 5: Advanced Networking & WebSockets (5 hours)
- Networking Architecture
- Building modular API clients
- Protocol-oriented network layers
- Router patterns for endpoints
- Advanced Request Handling
- Interceptors and middleware
- Authentication token management
- Offline-first strategies
- WebSockets & Real-time Communication
- WebSocket implementation
- Socket.IO integration
- Push notification advanced techniques
- GraphQL Introduction
- GraphQL vs REST
- Query construction
- Apollo client integration
Module 6: Security & Compliance (4 hours)
- Data Security
- Keychain Services in depth
- Secure enclave utilization
- Biometric authentication
- Network Security
- Certificate pinning
- HTTPS implementation details
- Man-in-the-middle attack prevention
- App Privacy
- App Transport Security configuration
- Privacy manifest implementation
- User data minimization
- Compliance Frameworks
- GDPR requirements for mobile apps
- HIPAA considerations (for healthcare)
- App Store privacy guidelines
Module 7: Unit Testing & CI/CD (4 hours)
- Advanced Testing Approaches
- Test-driven development workflows
- Behavior-driven development
- Snapshot testing for UI
- Mocking & Stubbing
- Network request mocking
- Dependency injection for testability
- Protocol-based mock objects
- Continuous Integration
- GitHub Actions for iOS
- Bitrise workflow configuration
- Fastlane automation scripts
- Continuous Delivery
- TestFlight automation
- Beta distribution strategies
- Release train management
Module 8: Production-Ready App Project (10-12 hours)
- Project Implementation
- Requirements analysis and architecture selection
- UI/UX implementation following Apple guidelines
- Core functionality development
- Testing suite creation
- Project Options
- E-commerce application
- Media streaming platform
- Enterprise data management tool
- Social networking app
- Production Considerations
- App Store optimization
- Analytics integration
- Crash reporting setup
- User feedback mechanisms
Learning Resources
- Advanced Swift coding examples
- Architecture template projects
- Networking and security sample implementations
- CI/CD configuration templates
- Comprehensive documentation and reference guides
Course Delivery
- Expert-led video tutorials
- Live coding sessions
- Architecture review workshops
- Group code review exercises
- 1-on-1 mentoring for project development
Program Benefits
- Master advanced iOS development techniques
- Implement production-grade architectural patterns
- Build secure and performant iOS applications
- Establish efficient development workflows
- Create a showcase project for career advancement
- Prepare for senior iOS developer positions
Estimated Cost (INR)
- Professional Package: ₹65,000 – ₹75,000
- Full access to all advanced modules
- Code architecture review sessions
- Personalized career advancement roadmap
- Mock technical interviews for senior positions
Prerequisites
- 3-6 years of iOS development experience
- Strong foundation in Swift programming
- Familiarity with UIKit and iOS fundamentals
- Experience shipping at least one iOS application
- Mac with latest macOS and Xcode versions
Time Commitment
- Total course duration: 40-45 hours of content
- Recommended completion time: 6-8 weeks (at 6-8 hours per week)
- Elevate your iOS development skills to the senior level! Enroll now and master the advanced techniques needed for building professional, production-ready applications.