Program Overview
This comprehensive iOS development course is designed for beginners and junior developers with 0-3 years of experience. Through structured learning modules and hands-on projects, you’ll master the fundamentals of Swift programming and iOS app development, giving you the skills to create your own functional iOS applications.
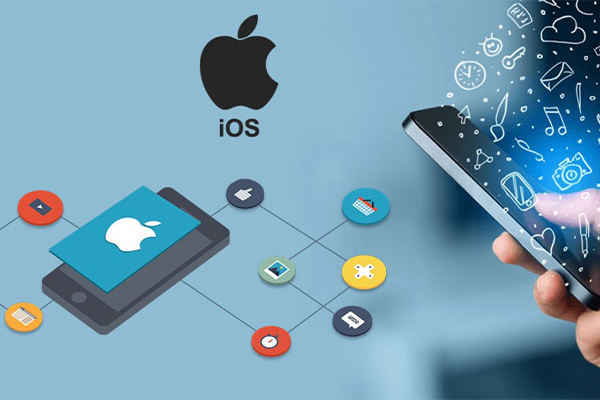
Detailed Curriculum
Module 1: Introduction to iOS Development (2.5 hours)
- iOS Development Environment
- Setting up Xcode
- iOS Simulator basics
- Apple Developer account overview
- iOS Architecture
- Understanding iOS platforms (iPhone, iPad)
- iOS version history and compatibility
- App Store guidelines and submission process
- Development Fundamentals
- MVC design pattern in iOS
- App lifecycle
- iOS project structure
Module 2: Swift Programming Basics (5 hours)
- Swift Fundamentals
- Variables, constants, and data types
- Optionals and unwrapping
- Control flow (if-else, switch, loops)
- Functions and Closures
- Function declarations and parameters
- Return types and multiple returns
- Closure syntax and usage
- Object-Oriented Programming
- Classes, structs, and enums
- Properties and methods
- Inheritance and protocols
- Access control and memory management
Module 3: User Interface & Storyboards (5 hours)
- UI Basics
- UIKit components overview
- Auto Layout and constraints
- Creating responsive interfaces
- View Controllers
- Understanding view controller lifecycle
- Navigation between screens
- Tab Bar and Navigation controllers
- Custom UI Components
- Creating custom views
- Table and collection views
- Gesture recognizers
Module 4: Networking & APIs (4 hours)
- HTTP Networking
- URLSession and HTTP requests
- Working with JSON data
- Asynchronous programming
- API Integration
- RESTful API concepts
- Authentication methods
- Error handling in network requests
- Practical Application
- Weather app or movie database integration
- Displaying remote images
- Caching network responses
Module 5: Data Persistence (4 hours)
- Local Storage Options
- UserDefaults for simple data
- File system operations
- Property lists and JSON serialization
- Core Data Framework
- Core Data architecture
- Data modeling
- CRUD operations
- Relationships and migrations
- Third-Party Solutions
- Introduction to Realm
- SQLite basics
- Choosing the right persistence solution
Module 6: Debugging & Optimization (2.5 hours)
- Debugging Techniques
- Using Xcode debugger
- Breakpoints and console
- Memory and performance profiling
- Code Optimization
- Memory management best practices
- UI performance improvements
- Battery and resource optimization
- Testing
- Unit testing basics
- UI testing introduction
- Test-driven development concepts
Module 7: Mini Project (7-10 hours)
- End-to-End App Development
- Requirements and planning
- UI/UX design implementation
- Feature development
- Testing and debugging
- Final Deployment
- Preparing for App Store submission
Learning Resources
- Comprehensive lecture videos and presentations
- Code examples and starter projects
- Swift documentation and reference guides
- Xcode tips and shortcuts guide
- Design resources and UI component templates
Course Delivery
- On-demand video tutorials
- Interactive coding exercises
- Weekly live Q&A sessions
- Peer code reviews
- Instructor feedback on projects
Program Benefits
- Build a strong foundation in Swift programming
- Develop functional iOS applications from scratch
- Understand iOS design patterns and best practices
- Create a portfolio-worthy project
- Prepare for junior iOS developer roles
- Receive a course completion certificate
Estimated Cost (INR)
- Complete Package: ₹35,000 – ₹40,000
- Full access to all course modules
- Mini-project guidance and review
- 1-on-1 mentoring sessions (3 sessions)
- Code reviews and personalized feedback
- Career guidance and interview preparation
- Resume and portfolio review
- iOS development resources library
Prerequisites
- Basic programming knowledge (helpful but not required)
- Mac computer with macOS Monterey or later
- Xcode 13 or newer
- No prior Swift or iOS experience needed
Time Commitment
- Total course duration: 30-35 hours of content
- Recommended completion time: 4-5 weeks (at 7-8 hours per week)